
Introduction
What is the most important thing to create a building? Strong base. The same rules apply to the framework to create automation testing.
Below is a class called base class, which contains the driver for Selenium. It also listens to data.properties classes, so it can execute code for different browsers such as Chrome, Firefox, and Internet Explorer based on the information provided in those classes. Due to time constraints, I did not add Firefox or Internet Explorer drivers.
Guess what? There is also a screenshot feature that returns its location. Just pay attention to the last method.
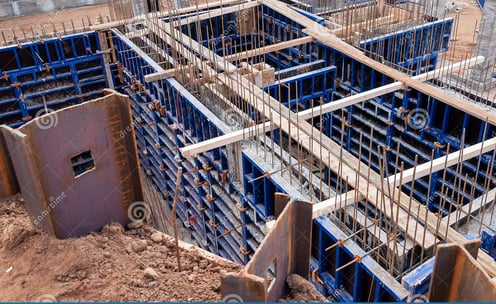
package resources;
import org.apache.logging.log4j.core.util.FileUtils;
import org.openqa.selenium.OutputType;
import org.openqa.selenium.TakesScreenshot;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.io.FileHandler;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.util.Properties;
import java.util.concurrent.TimeUnit;
public class base {
public WebDriver driver;
public Properties prop;
public WebDriver initializedDriver() throws IOException {
prop = new Properties();
FileInputStream file=new FileInputStream("/Users/muradnabizade/Desktop/EndToEndAuto/E2EProject/src/main/java/resources/data.properties");
prop.load(file);
String browserName= prop.getProperty("browser");
if(browserName.equals("chrome")){
//execute in chrome driver
System.setProperty("webdriver.chrome.driver", "/Users/muradnabizade/Downloads/chromedriver 2");
driver=new ChromeDriver();
} else if (browserName.equals("firefox")) {
//FIREFOX DRIVER can be added here
}
else if(browserName.equals("IE")){
//IE driver can be added here
}
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
return driver;
}
public String getScreenShotPath(String testCaseName, WebDriver driver) throws IOException {
TakesScreenshot ts=(TakesScreenshot) driver;
File source = ts.getScreenshotAs(OutputType.FILE);
String destinationFile=System.getProperty("user.dir")+"/reports2/"+testCaseName+".png";
FileHandler.copy(source, new File(destinationFile));
return destinationFile;
}
}
data.properties
Simple file to store data such as browser name or URL.
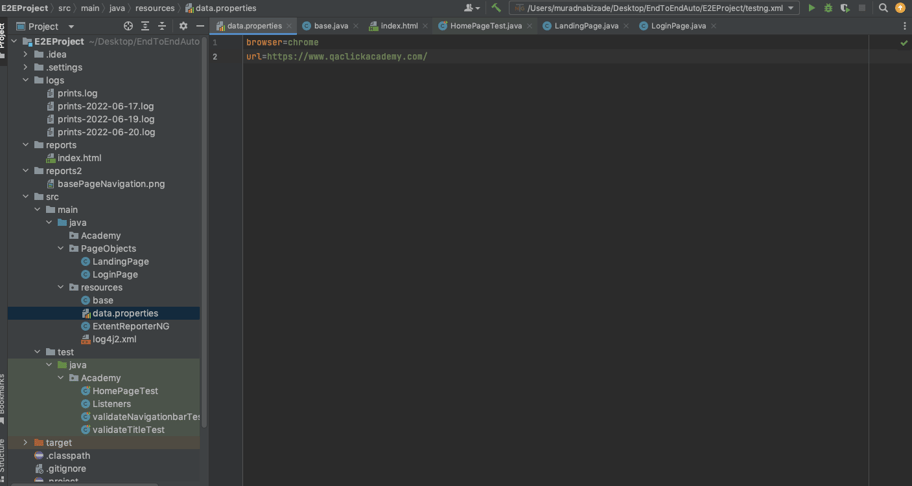
Big LIE
How I can build base?
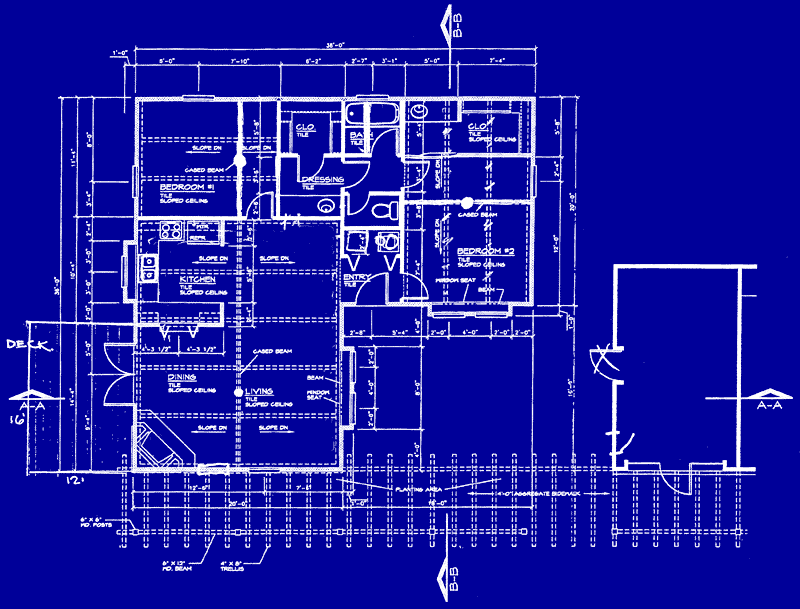
Yes, I might be wrong when I say the most important thing to creating a building is a base. Why? How you can even create a base without a plan? Or How can you know that workers will do their job? We need blueprints and management tools.
No, we need magical tools. Let me introduce you to Maven and TestNG.
Maven is used to defining project structure, dependencies, build, and test management. Using pom. XML(Maven) you can configure dependencies needed for building testing and running code.
TestNG makes automated tests more structured, readable, maintainable, and user-friendly. For example, you can tell your TestNG to run test cases in parallel mode or take screenshots when a test is failed.
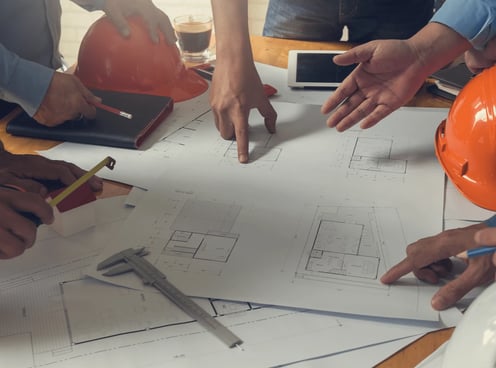
TESTNG
<p><?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd">
<suite name="All Test Suite" parallel="tests">
<listeners>
<listener class-name="Academy.Listeners"></listener>
</listeners>
<test verbose="2" preserve-order="true" name="/Users/muradnabizade/Desktop/EndToEndAuto/E2EProject">
<classes>
<class name="Academy.HomePageTest">
<methods>
<include name="basePageNavigation"/>
</methods>
</class>
</classes>
</test>
<test name="Navigation">
<classes>
<class name="Academy.validateNavigationbarTest">
<methods>
<include name="basePageNavigation"/>
</methods>
</class>
</classes>
</test>
<test name="Validate Title">
<classes>
<class name="Academy.validateTitleTest">
<methods>
<include name="basePageNavigation"/>
</methods>
</class>
</classes>
</test>
</suite></p>
Maven(pom.xml)
<p>
<dependencies>
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-api</artifactId>
<version>2.8.2</version>
</dependency>
<!--mvnrepository.com/artifact/org.apache.logging.log4j/log4j-1.2-api -->
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-1.2-api</artifactId>
<version>2.13.3</version>
<scope>test</scope>
</dependency>
<!--mvnrepository.com/artifact/org.apache.logging.log4j/log4j-1.2-api -->
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-1.2-api</artifactId>
<version>2.13.3</version>
<scope>test</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java -->
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.2.2</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.aventstack/extentreports -->
<dependency>
<groupId>com.aventstack</groupId>
<artifactId>extentreports</artifactId>
<version>5.0.9</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.testng/testng -->
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.6.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/commons-io/commons-io -->
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.11.0</version>
</dependency>
</dependencies></p>
LandingPage.java
The purpose of this class is to save information like the CSS path for the title, Navbar, etc. A get login function has been added so that it can be accessed outside of this class whenever needed.
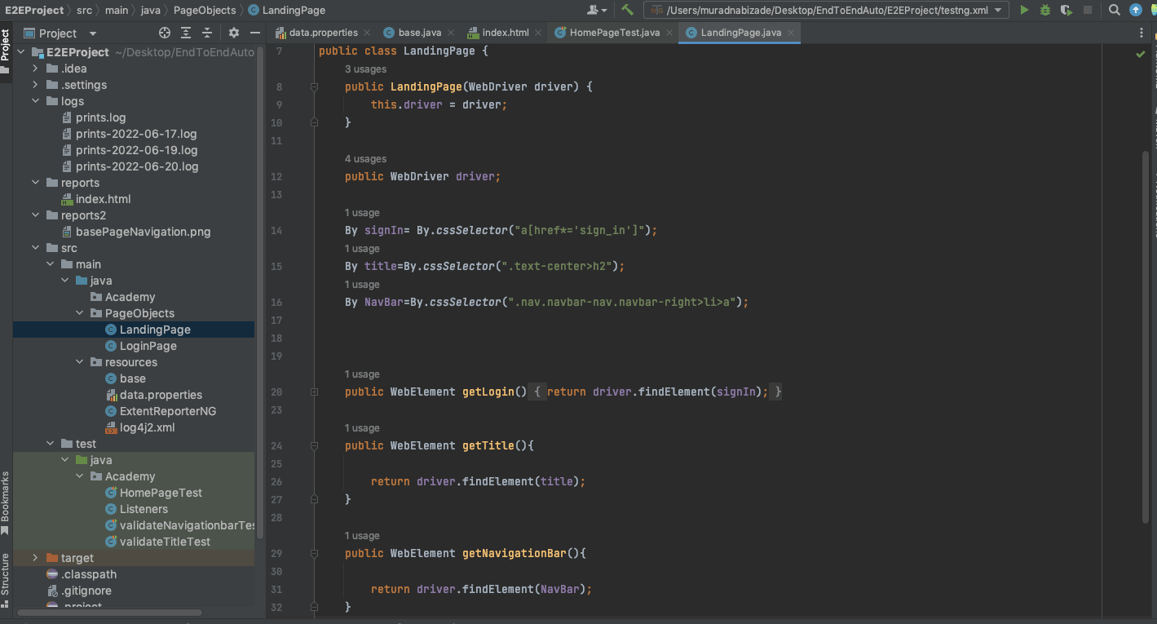
LoginPage.java
A very similar class is mentioned above but this time it is for the login page. This class will store page objects for the login page and find the sign-in button and it will enter the username and password.
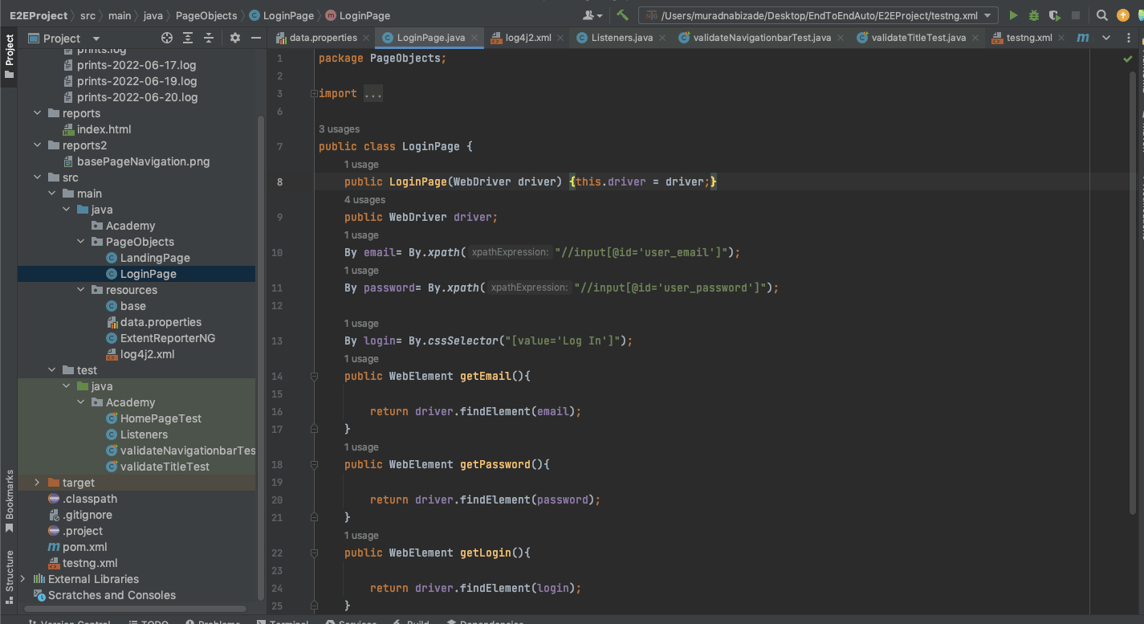
First test case
It uses page objects from landingPage.java class and sends username and password to the website. It uses the DataProvider function of Testing to send the data.
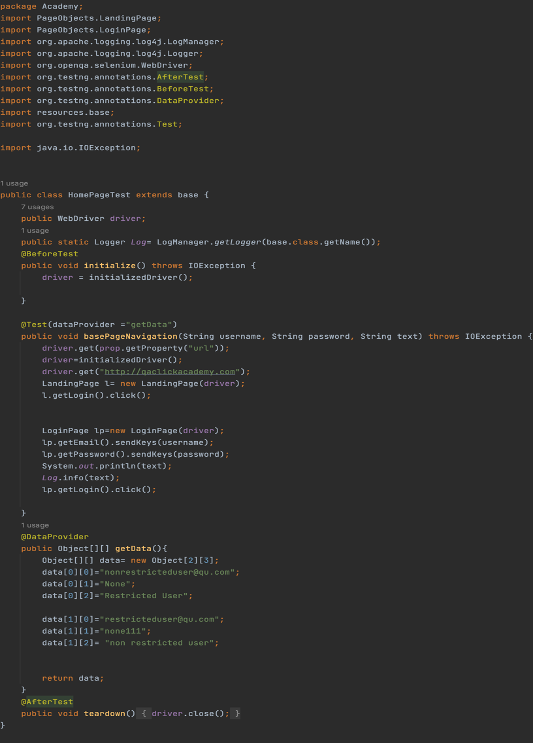
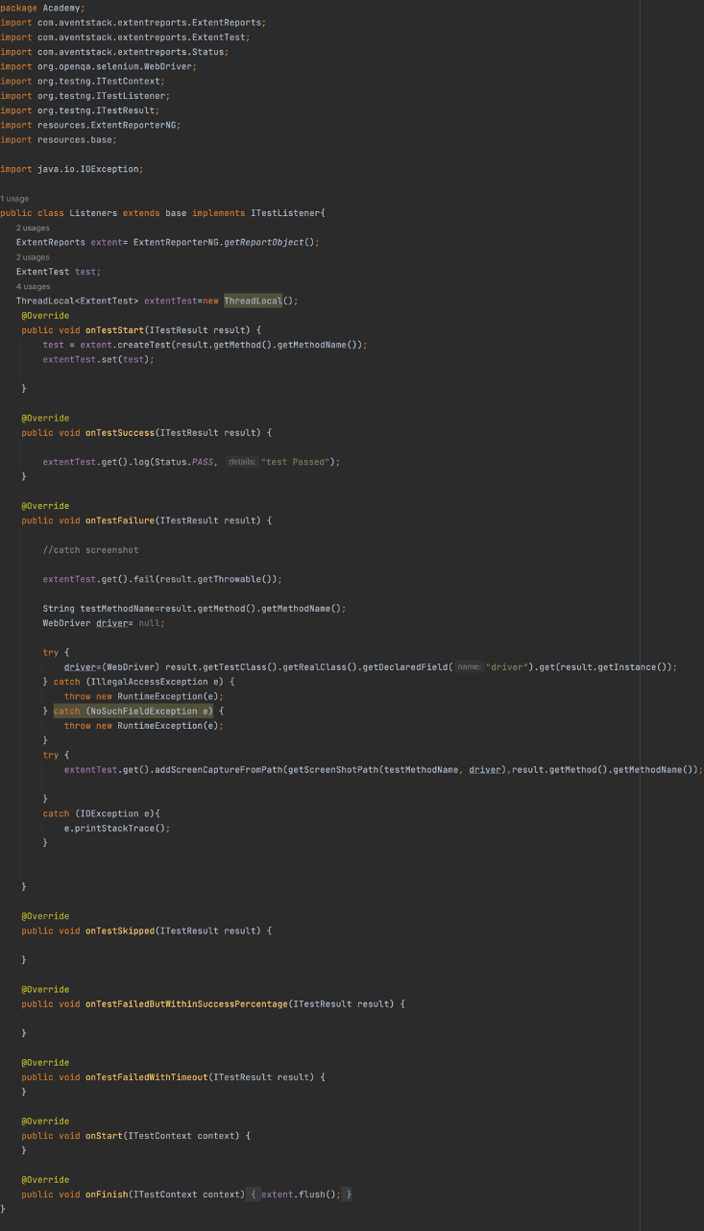
Listener class
This class is used to form the structure for test classes and tell what to do when the test failed or passed.
For example, it takes screenshots when a test is failed and adds log information to the report.
Report (ExtentReport)
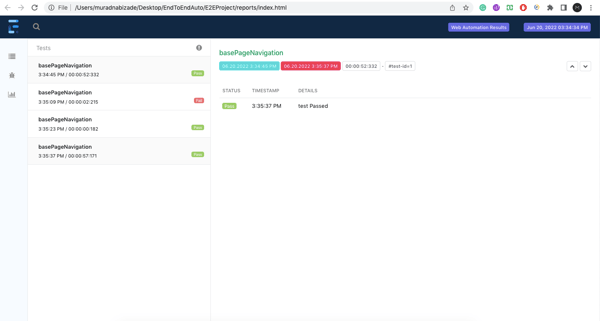
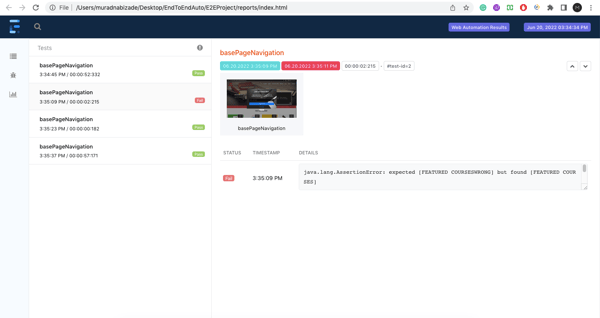
Please don't take any construction advice from me if you don't want to end up like this.
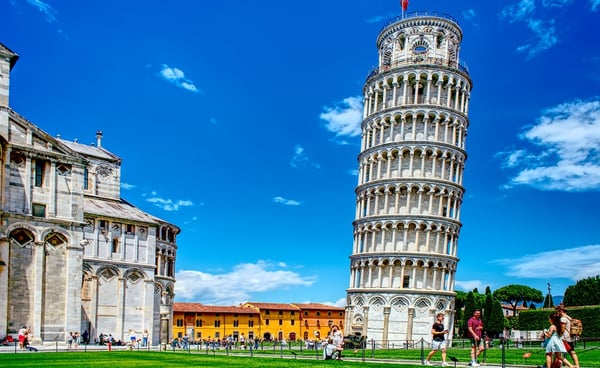
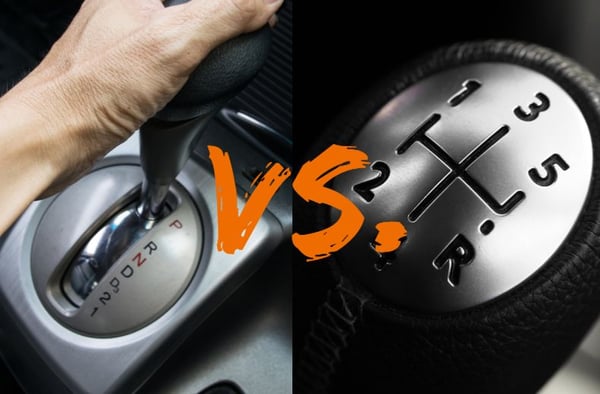
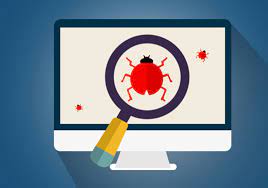